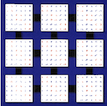 |
ScaLAPACK 2.1
2.1
ScaLAPACK: Scalable Linear Algebra PACKage
|
Go to the documentation of this file.
3 #if (INTFACE == C_CALL)
4 void Czgerv2d(
int ConTxt,
int m,
int n,
double *A,
int lda,
int rsrc,
int csrc)
51 void BI_ArgCheck(
int,
int,
char *,
char,
char,
char,
int,
int,
int,
int,
67 #if (BlacsDebugLvl > 0)
72 else tlda =
Mpval(lda);
F_VOID_FUNC zgerv2d_(int *ConTxt, int *m, int *n, double *A, int *lda, int *rsrc, int *csrc)
BLACBUFF * BI_GetBuff(int length)
void BI_Unpack(BLACSCONTEXT *ctxt, BVOID *A, BLACBUFF *bp, MPI_Datatype Dtype)
#define MGetConTxt(Context, ctxtptr)
#define Mkpnum(ctxt, prow, pcol)
MPI_Datatype BI_GetMpiGeType(BLACSCONTEXT *ctxt, int m, int n, int lda, MPI_Datatype Dtype, int *N)
void BI_Srecv(BLACSCONTEXT *ctxt, int src, int msgid, BLACBUFF *bp)
void BI_ArgCheck(int ConTxt, int RoutType, char *routine, char scope, char uplo, char diag, int m, int n, int lda, int nprocs, int *prows, int *pcols)
#define BI_MPI_TYPE_FREE(t)
void BI_UpdateBuffs(BLACBUFF *Newbp)
int BI_BuffIsFree(BLACBUFF *bp, int Wait)