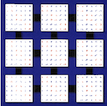 |
ScaLAPACK 2.1
2.1
ScaLAPACK: Scalable Linear Algebra PACKage
|
Go to the documentation of this file.
3 #if (INTFACE == C_CALL)
4 void Cdtrrv2d(
int ConTxt,
char *uplo,
char *diag,
int m,
int n,
double *A,
5 int lda,
int rsrc,
int csrc)
8 double *A,
int *lda,
int *rsrc,
int *csrc)
64 void BI_ArgCheck(
int,
int,
char *,
char,
char,
char,
int,
int,
int,
int,
73 int tuplo, tdiag, tlda;
86 #if (BlacsDebugLvl > 0)
91 else tlda =
Mpval(lda);
BLACBUFF * BI_GetBuff(int length)
void BI_Unpack(BLACSCONTEXT *ctxt, BVOID *A, BLACBUFF *bp, MPI_Datatype Dtype)
MPI_Datatype BI_GetMpiTrType(BLACSCONTEXT *ctxt, char uplo, char diag, int m, int n, int lda, MPI_Datatype Dtype, int *N)
#define MGetConTxt(Context, ctxtptr)
#define Mkpnum(ctxt, prow, pcol)
F_VOID_FUNC dtrrv2d_(int *ConTxt, F_CHAR uplo, F_CHAR diag, int *m, int *n, double *A, int *lda, int *rsrc, int *csrc)
void BI_Srecv(BLACSCONTEXT *ctxt, int src, int msgid, BLACBUFF *bp)
void BI_ArgCheck(int ConTxt, int RoutType, char *routine, char scope, char uplo, char diag, int m, int n, int lda, int nprocs, int *prows, int *pcols)
#define BI_MPI_TYPE_FREE(t)
void BI_UpdateBuffs(BLACBUFF *Newbp)
int BI_BuffIsFree(BLACBUFF *bp, int Wait)