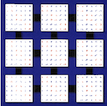 |
ScaLAPACK 2.1
2.1
ScaLAPACK: Scalable Linear Algebra PACKage
|
Go to the documentation of this file.
3 #if (INTFACE == C_CALL)
4 void Cctrbr2d(
int ConTxt,
char *scope,
char *top,
char *uplo,
char *diag,
5 int m,
int n,
float *A,
int lda,
int rsrc,
int csrc)
8 F_CHAR diag,
int *m,
int *n,
float *A,
int *lda,
71 void BI_ArgCheck(
int,
int,
char *,
char,
char,
char,
int,
int,
int,
int,
91 int length, src, tlda, error, one=1;
92 char ttop, tscope, tuplo, tdiag;
104 #if (BlacsDebugLvl > 0)
113 if (ttop ==
' ') ttop = DefBSTop;
116 else tlda =
Mpval(m);
161 MPI_Pack_size(one, MatTyp, ctxt->
scp->
comm, &length);
164 bp->
dtype = MPI_PACKED;
166 if (MatTyp == MPI_BYTE)
170 bp->
dtype = MPI_BYTE;
179 error =
BI_HypBR(ctxt, bp, send, src);
BLACBUFF * BI_GetBuff(int length)
void BI_Unpack(BLACSCONTEXT *ctxt, BVOID *A, BLACBUFF *bp, MPI_Datatype Dtype)
MPI_Datatype BI_GetMpiTrType(BLACSCONTEXT *ctxt, char uplo, char diag, int m, int n, int lda, MPI_Datatype Dtype, int *N)
void BI_Asend(BLACSCONTEXT *ctxt, int dest, int msgid, BLACBUFF *bp)
void BI_SringBR(BLACSCONTEXT *ctxt, BLACBUFF *bp, SDRVPTR send, int src)
void BI_Ssend(BLACSCONTEXT *ctxt, int dest, int msgid, BLACBUFF *bp)
void BI_MpathBR(BLACSCONTEXT *ctxt, BLACBUFF *bp, SDRVPTR send, int src, int npaths)
#define MGetConTxt(Context, ctxtptr)
int BI_HypBR(BLACSCONTEXT *ctxt, BLACBUFF *bp, SDRVPTR send, int src)
void BI_IdringBR(BLACSCONTEXT *ctxt, BLACBUFF *bp, SDRVPTR send, int src, int step)
void BI_TreeBR(BLACSCONTEXT *ctxt, BLACBUFF *bp, SDRVPTR send, int src, int nbranches)
F_VOID_FUNC ctrbr2d_(int *ConTxt, F_CHAR scope, F_CHAR top, F_CHAR uplo, F_CHAR diag, int *m, int *n, float *A, int *lda, int *rsrc, int *csrc)
void(* SDRVPTR)(BLACSCONTEXT *, int, int, BLACBUFF *)
void BI_BlacsErr(int ConTxt, int line, char *file, char *form,...)
void BI_ArgCheck(int ConTxt, int RoutType, char *routine, char scope, char uplo, char diag, int m, int n, int lda, int nprocs, int *prows, int *pcols)
#define BI_MPI_TYPE_FREE(t)
#define Mvkpnum(ctxt, prow, pcol)
void BI_UpdateBuffs(BLACBUFF *Newbp)
int BI_BuffIsFree(BLACBUFF *bp, int Wait)